Exploring Databases In JavaScript With Local Storage
4.9 out of 5 based on 10579 votesLast updated on 28th Nov 2024 13.85K Views
- Bookmark
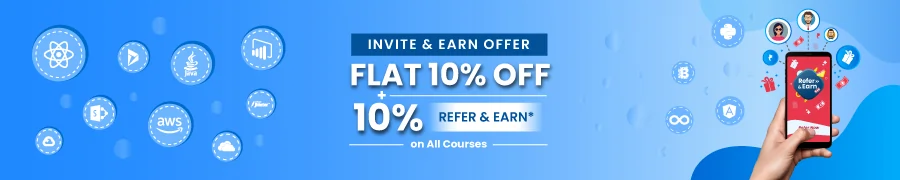
Learn how to use Local Storage in JavaScript to store data persistently on the user's browser with simple key-value pairs for web apps.
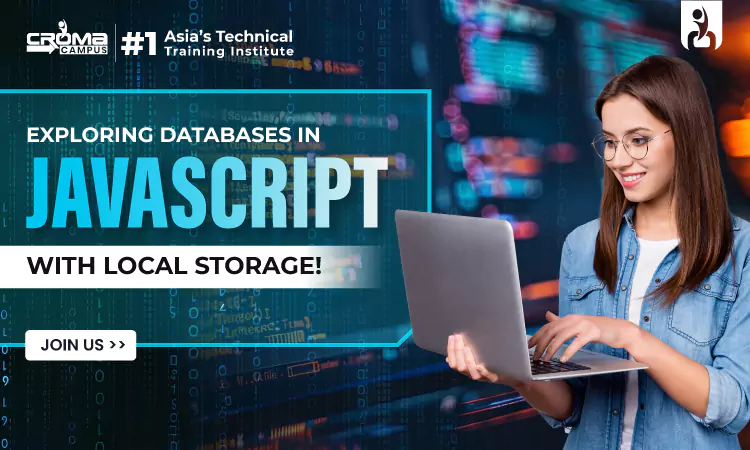
Overview
When building websites or web applications, you often need to store data. For example, you might want to save user preferences, remember items in a shopping cart, or store form data temporarily. Traditionally, this data is stored in a server-side database, like MySQL or MongoDB. But did you know you can store data right in the user's web browser using Local Storage in JavaScript? This can be very useful for small projects or when you want to store simple data without the need for a back-end server. If you’re new to this, taking a JavaScript Online Course can help you learn how to use Local Storage and other JavaScript tools.
Local Storage is part of the Web Storage API and allows websites to store data in a browser with no expiration date. This is different from cookies, which can only hold small amounts of data (usually 4KB). Local Storage can store around 5-10MB of data, depending on the browser. The best part? The data remains even when the browser is closed and reopened, making it a great choice for saving user preferences or session data.
In this blog, we will explore how to use Local Storage in JavaScript. We will look at how to store, retrieve, and delete data, as well as how you can use it in practical scenarios. We will also discuss some best practices and limitations to keep in mind when working with Local Storage.
What is Local Storage?
Local Storage is a feature of modern web browsers that allows websites to store data on the user’s device. This data is stored as key-value pairs, where each key is a unique identifier for the data, and the value is the actual data stored.
For example, a website could store a user’s language preference, theme setting (dark or light mode), or even a list of items in their shopping cart. The data is stored in the browser and can be accessed by the website as long as the user does not clear their browser cache.
Unlike cookies, which expire after a certain time, Local Storage keeps the data indefinitely until you manually delete it. This makes it perfect for storing things like user preferences or session data that should persist even if the user leaves and comes back later. For those interested in further exploring web development and enhancing their skills, taking a Web Development Online Course or a Web Development Course in Delhi can deepen your understanding of JavaScript and Local Storage.
How to Use Local Storage in JavaScript?
Using Local Storage in JavaScript is simple and straightforward. There are just a few basic methods to know: setItem(), getItem(), removeItem(), and clear(). Let’s break these down and look at examples of how to use them.
1. Storing Data in Local Storage
To store data, you use the setItem() method. This method takes two arguments: a key (the name of the data) and the value (the data itself).
For example, let’s say you want to store a user’s theme preference (light or dark mode):
javascript
Copy code
localStorage.setItem('theme', 'dark');
This stores the key 'theme' with the value 'dark' in Local Storage. If you want to store an object, you need to first convert it into a string using JSON.stringify().
For example, to store an object:
javascript
Copy code
let user = { name: "John", age: 30 };
localStorage.setItem('user', JSON.stringify(user));
Here, the user object is converted into a string before being stored.
2. Retrieving Data from Local Storage
This method takes one argument: the key, and it returns the value associated with that key.
For example:
javascript
Copy code
let theme = localStorage.getItem('theme');
console.log(theme); // Outputs: dark
If the key does not exist, getItem() will return null, so you should always check for that before using the data.
To retrieve and use the user object we saved earlier:
javascript
Copy code
let user = JSON.parse(localStorage.getItem('user'));
console.log(user.name); // Outputs: John
In this case, we used JSON.parse() to convert the string back into an object.
3. Removing Data from Local Storage
If you want to remove a specific item from Local Storage, you can use the removeItem() method. This method takes the key of the item you want to remove.
javascript
Copy code
localStorage.removeItem('theme');
This will delete the item with the key 'theme' from Local Storage.
Tips: Java is a powerful programming language used for web, mobile, and enterprise applications. Enrolling in Java Training in Noida enhances coding skills, career opportunities, and industry-relevant expertise.
4. Clearing All Data from Local Storage
If you want to clear all data stored in Local Storage, you can use the clear() method. This removes everything stored in Local Storage for your website.
javascript
Copy code
localStorage.clear();
This will delete all key-value pairs from Local Storage.
Also Read This:
JavaScript Interview Questions
Web Development Course Syllabus
Web Development Interview Questions
Note: Django is a high-level Python web framework enabling rapid development and clean design. Enroll in a Django Course in Noida to master building robust, scalable web applications efficiently.
Practical Uses of Local Storage
Now that we understand the basics of using Local Storage, let's look at some real-world scenarios where it can be helpful. Local Storage can be used to save a variety of data, including user preferences, session data, and even items in a shopping cart.
1. Saving User Preferences
One common use of Local Storage is saving user preferences. For example, if your website offers a choice between light and dark mode, you can use Local Storage to remember the user’s theme choice.
Here’s an example:
javascript
Copy code
// Save theme preference
function setTheme(theme) {
localStorage.setItem('theme', theme);
document.body.className = theme;
}
// Load theme preference
function loadTheme() {
const theme = localStorage.getItem('theme');
if (theme) {
document.body.className = theme;
}
}
loadTheme(); // Load theme when the page loads
In this example, when the user selects a theme, the choice is saved in Local Storage. When the page is reloaded, the theme is applied based on the saved preference.
2. Saving Form Data
Another common use case is saving form data. If the user is filling out a form and accidentally reloads the page, you can save the form data to Local Storage and reload it when the page is refreshed. This prevents the user from losing their progress.
// Save form data
document.getElementById('myForm').addEventListener('input', function() {
const formData = new FormData(this);
localStorage.setItem('formData', JSON.stringify(Object.fromEntries(formData)));
});
// Load form data
document.addEventListener('DOMContentLoaded', function() {
const savedData = JSON.parse(localStorage.getItem('formData'));
if (savedData) {
for (let key in savedData) {
document.querySelector(`[name=${key}]`).value = savedData[key];
}
}
});
In this example, every time the user types in a form field, the data is saved to Local Storage. When the page reloads, the data is restored to the form fields.
3. Saving Cart Items in E-commerce Sites
For e-commerce websites, Local Storage is perfect for saving the items in a user’s shopping cart. Even if the user closes their browser or navigates away, their cart will still be intact when they return.
javascript
Copy code
// Add item to cart
function addToCart(product) {
let cart = JSON.parse(localStorage.getItem('cart')) || [];
cart.push(product);
localStorage.setItem('cart', JSON.stringify(cart));
}
// Get cart items
function getCart() {
return JSON.parse(localStorage.getItem('cart')) || [];
}
In this example, when a product is added to the cart, it is saved to Local Storage. When the user revisits the site, the cart is reloaded with the saved items.
Note: The MEAN Stack, consisting of MongoDB, Express.js, Angular, and Node.js, is a powerful web development framework. Enrolling in a MEAN Stack Course will help you master full-stack JavaScript development.
Best Practices and Limitations
While Local Storage is a useful tool, it does have some limitations and best practices to keep in mind.
Limitations
- Storage Limits: Local Storage can only hold around 5-10MB of data, which is fine for simple data but not suitable for storing large files or databases.
- Security: Local Storage is not encrypted, meaning that any sensitive data should not be stored there. For example, don’t store passwords or credit card information in Local Storage.
- Synchronous API: Local Storage operations are synchronous, which means that they can block other operations, especially if you’re working with large data.
- Browser Compatibility: Some older browsers may not support Local Storage, so you should always check for support before using it in production.
Best Practices
- Use JSON: Since Local Storage only stores strings, always use JSON.stringify() to store objects and JSON.parse() to retrieve them.
- Check for Availability: Not all browsers support Local Storage. Always check if it is available before using it.
Similarly, for those interested in the visual side of web development, courses like Web Designing Online Training or Web Designing Training in Delhi provide valuable insights into creating user-friendly websites.
Relevant Web Development Courses:
Node JS Full Stack Developer Course
Playwright Automation with JS Course
Advanced Python Programming Course
Full Stack Developer in Python Course
Sum up,
Local Storage is a powerful tool for storing data on the client-side in JavaScript. It is easy to use and perfect for saving user preferences, form data, or shopping cart contents. While it has some limitations, such as storage size and security concerns, it is still a great option for small to medium-sized projects.
By understanding how Local Storage works and combining it with other web technologies, you can build more interactive and dynamic web applications.
Subscribe For Free Demo
Free Demo for Corporate & Online Trainings.
Your email address will not be published. Required fields are marked *
Course Features





